Running A Pi Pie Chart turned out some useful performance numbers. It’s almost, but not quite, a Raspberry Pi 3B in a Raspberry Pi Zero form factor.
32-bit mode
Running stock Raspberry Pi OS with desktop, compiled with stock options:
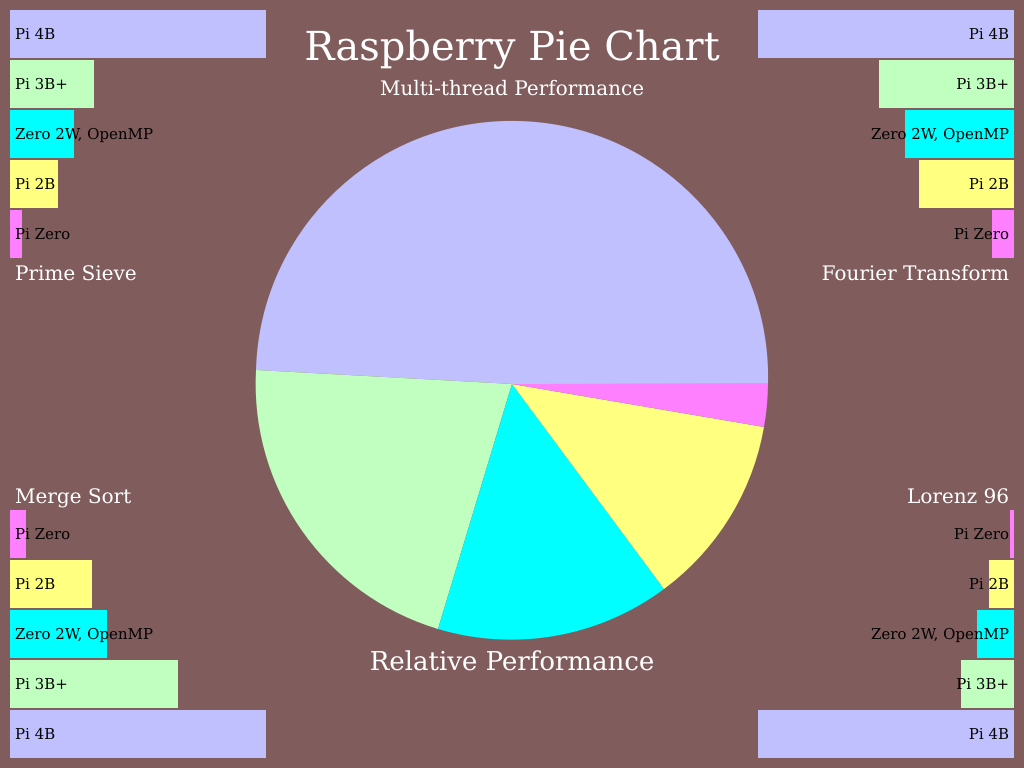
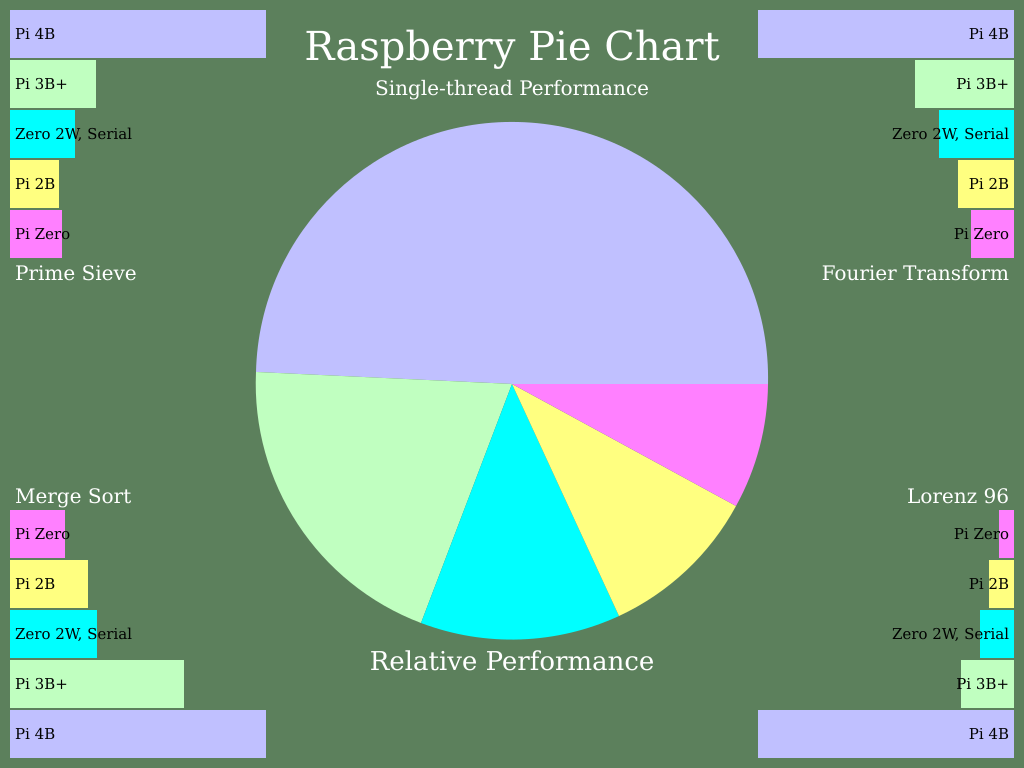
time ./pichart-openmp -t "Zero 2W, OpenMP" pichart -- Raspberry Pi Performance OPENMP version 36 Prime Sieve P=14630843 Workers=4 Sec=2.18676 Mops=427.266 Merge Sort N=16777216 Workers=8 Sec=1.9341 Mops=208.186 Fourier Transform N=4194304 Workers=8 Sec=3.10982 Mflops=148.36 Lorenz 96 N=32768 K=16384 Workers=4 Sec=4.56845 Mflops=705.102 The Zero 2W, OpenMP has Raspberry Pi ratio=8.72113 Making pie charts...done. real 8m20.245s user 15m27.197s sys 0m3.752s ----------------------------- time ./pichart-serial -t "Zero 2W, Serial" pichart -- Raspberry Pi Performance Serial version 36 Prime Sieve P=14630843 Workers=1 Sec=8.77047 Mops=106.531 Merge Sort N=16777216 Workers=2 Sec=7.02049 Mops=57.354 Fourier Transform N=4194304 Workers=2 Sec=8.58785 Mflops=53.724 Lorenz 96 N=32768 K=16384 Workers=1 Sec=17.1408 Mflops=187.927 The Zero 2W, Serial has Raspberry Pi ratio=2.48852 Making pie charts...done. real 7m50.524s user 7m48.854s sys 0m1.370s
64-bit
Running stock/beta 64-bit Raspberry Pi OS with desktop. Curiously, these ran out of memory (at least, in oom-kill‘s opinion) with the desktop running, so I had to run from console. This also meant it was harder to capture the program run times.
The firmware required to run in this mode should be in the official distribution by now.
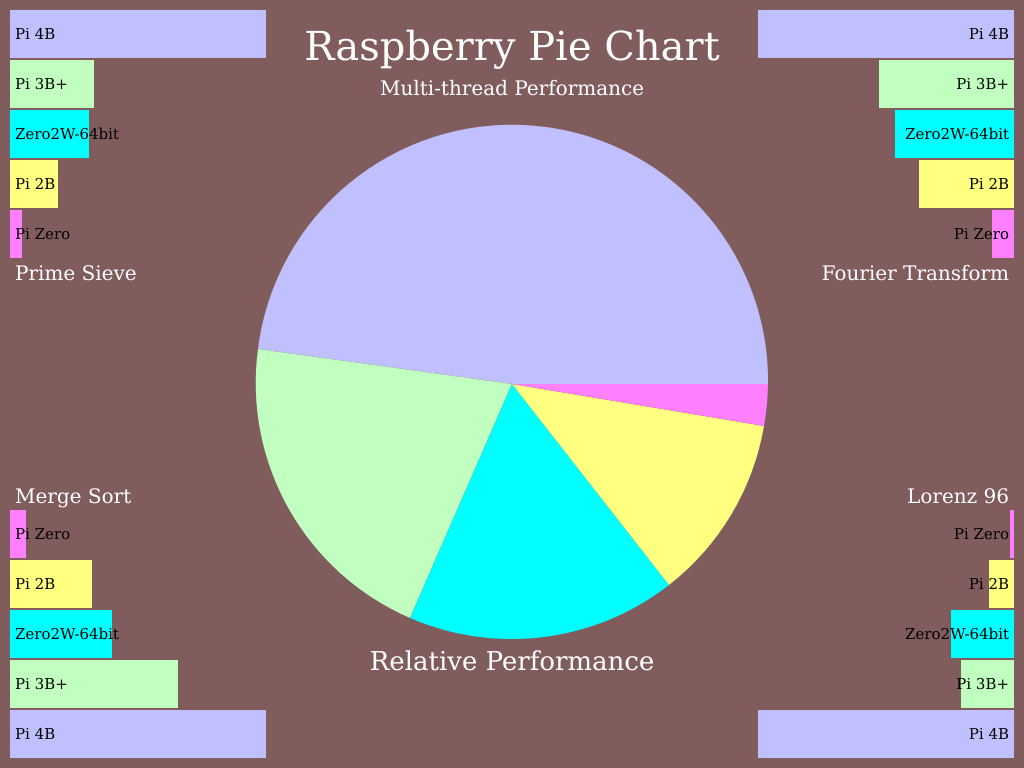
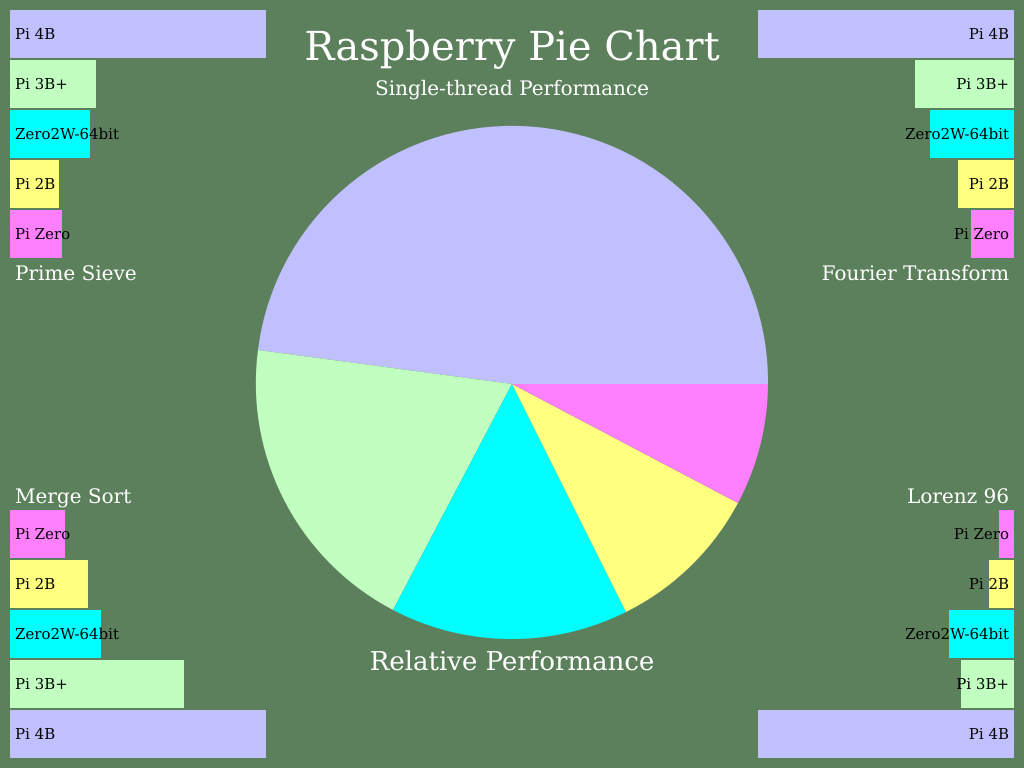
pichart -- Raspberry Pi Performance OPENMP version 36 Prime Sieve P=14630843 Workers=4 Sec=1.78173 Mops=524.395 Merge Sort N=16777216 Workers=8 Sec=1.83854 Mops=219.007 Fourier Transform N=4194304 Workers=4 Sec=2.83797 Mflops=162.572 Lorenz 96 N=32768 K=16384 Workers=4 Sec=2.66808 Mflops=1207.32 The Zero2W-64bit has Raspberry Pi ratio=10.8802 Making pie charts...done. ------------------------- pichart -- Raspberry Pi Performance Serial version 36 Prime Sieve P=14630843 Workers=1 Sec=7.06226 Mops=132.299 Merge Sort N=16777216 Workers=2 Sec=6.75762 Mops=59.5851 Fourier Transform N=4194304 Workers=2 Sec=7.73993 Mflops=59.6095 Lorenz 96 N=32768 K=16384 Workers=1 Sec=9.00538 Mflops=357.7 The Zero2W-64bit has Raspberry Pi ratio=3.19724 Making pie charts...done.
The main reason for the Raspberry Pi Zero 2 W appearing slower than the 3B and 3B+ is likely that it uses LPDDR2 memory instead of LPDDR3. 64-bit mode provides is a useful performance increase, offset by increased memory use. I found desktop apps to be almost unusably swappy in 64-bit mode, but there might be some tweaking I can do to avoid this.
Unlike the single core Raspberry Pi Zero, the Raspberry Pi Zero 2 W can be made to go into thermal throttling if you’re really, really determined. Like “3 or more cores running flat-out“-determined. In my testing, two cores at 100% (as you might get in emulation) won’t put it into thermal throttling, even in the snug official case closed up tight. More on this later.
(And a great big raspberry blown at Make, who leaked the Raspberry Pi Zero 2 W release a couple of days ago. Not classy.)